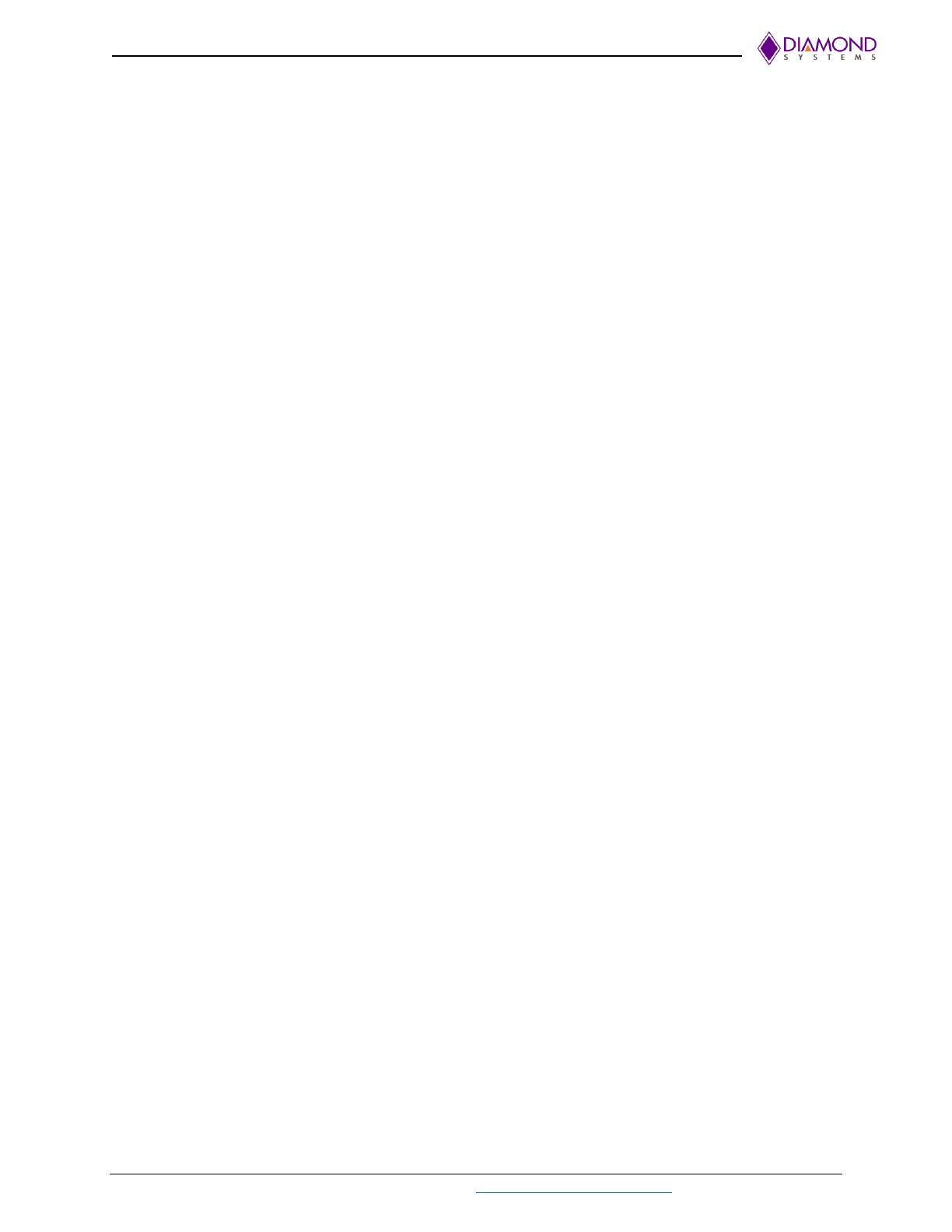
DS-MPE-GPIO Universal Software User Manual Rev A.0 www.diamondsystems.com Page 2
CONTENTS
1. Introduction ...................................................................................................................................................... 3
2. Hardware overview ........................................................................................................................................... 3
2.1 Description .................................................................................................................................................... 3
2.2 Specifications ................................................................................................................................................ 3
3. General programming guidelines ................................................................................................................... 4
3.1 Initialization and exit function calls ................................................................................................................ 4
3.2 Error handling ................................................................................................................................................ 5
4. Universal Driver API Description .................................................................................................................... 6
4.1 MPEGPIODIOConfig ..................................................................................................................................... 6
4.2 MPEGPIODIOConfigAll................................................................................................................................. 7
4.3 MPEGPIODIOOutputByte ............................................................................................................................. 7
4.4 MPEGPIODIOInputByte ................................................................................................................................ 8
4.5 MPEGPIODIOOutputBit ................................................................................................................................ 9
4.6 MPEGPIODIOInputBit ................................................................................................................................. 10
4.7 MPEGPIOCounterSetRate ......................................................................................................................... 11
4.8 MPEGPIOCounterConfig ............................................................................................................................ 12
4.9 MPEGPIOCounterRead .............................................................................................................................. 13
4.10 MPEGPIOCounterFunction ......................................................................................................................... 14
4.11 MPEGPIOCounterReset ............................................................................................................................. 15
4.12 MPEGPIOPWMConfig ................................................................................................................................ 16
4.13 MPEGPIOPWMStart ................................................................................................................................... 17
4.14 MPEGPIOPWMStop ................................................................................................................................... 17
4.15 MPEGPIOPWMCommand .......................................................................................................................... 18
4.16 MPEGPIOPWMReset ................................................................................................................................. 19
4.17 MPEGPOUserInterruptConfig ..................................................................................................................... 20
4.18 MPEGPIOUserInterruptRun ........................................................................................................................ 21
4.19 MPEGPIOUserInterruptCancel ................................................................................................................... 21
4.20 MPEGPIOInitBoard ..................................................................................................................................... 22
4.21 MPEGPIOFreeBoard .................................................................................................................................. 22
4.22 MPEGPIOLED ............................................................................................................................................ 23
4.23 MPEGPIOConfig ......................................................................................................................................... 23
5. Universal Driver Application ......................................................................................................................... 24
5.1 DIO .............................................................................................................................................................. 24
5.2 Counter........................................................................................................................................................ 25
5.3 PWM............................................................................................................................................................ 26
5.4 User Interrupt .............................................................................................................................................. 27
6. Common Task Reference .............................................................................................................................. 28
6.1 Feature Overview ........................................................................................................................................ 28
6.2 Digital I/O Software Task Reference ........................................................................................................... 30
6.3 Performing Digital IO Operations ................................................................................................................ 34
6.4 Performing Counter Operation .................................................................................................................... 36
6.5 Performing PWM operation ......................................................................................................................... 38
7. I/O connectors ................................................................................................................................................ 39
7.1 DS-MPE-GPIO Digital GPIO Connector ..................................................................................................... 39
8. Appendix: Reference Information ................................................................................................................ 40