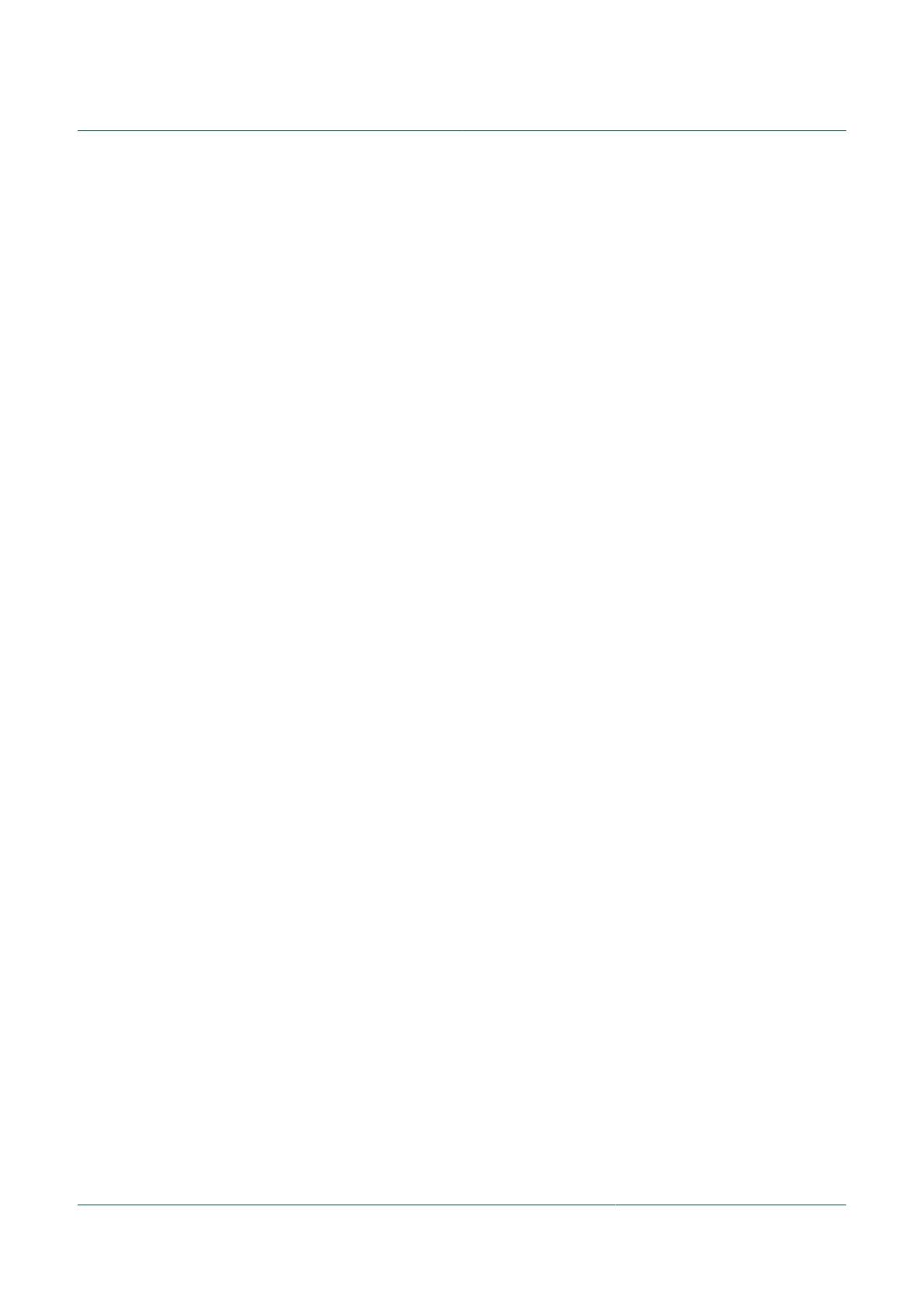
NXP Semiconductors
PT2001SWUG
PT2001 programming guide and instruction set
PT2001SWUG All information provided in this document is subject to legal disclaimers. © NXP B.V. 2019. All rights reserved.
User guide Rev. 3.0 — 29 April 2019
4 / 153
considered to be unsigned, so they are saturated between FFFFh (65535) and 0000h
(0).
– MASK_MAX bit is set if the result of the last mask operation is FFFFh.
– MASK_MIN bit is set if the result of the last mask operation is 0000h.
– MUL_SHIFT_OVR is set to 0 if the 16 MSBs of the last multiplication or 32-bit shift
result are all 0, otherwise it is 1.
– MUL_SHIFT_LOSS is set to 0 if the 16 LSBs of the last multiplication or 32-bit shift
result are all 0, otherwise it is 1.
– RES_ZERO is set if the result of the last addition or subtraction is zero.
– RES_SIGN is set if the result of the last addition or subtraction is negative.
– UNSIGNED_UND is set if the last addition or subtraction produced underflow,
considering the operands as unsigned numbers.
– SIGNED_UND is set if the last addition or subtraction produced underflow,
considering the operands as two's complement numbers.
– UNSIGNED_OVR is set if the last addition or subtraction produced overflow,
considering the operands as unsigned numbers.
– SIGNED_OVR is set if the last addition or subtraction produced overflow, considering
the operands as two's complement numbers.
The OP_DONE can be set by the ALU, and the Instruction_decoder can only read it. This
bit is set to 0 when a multicycle operation is in progress, otherwise is set to 1. If an ALU
operation is issued when another operation is in progress (that is when the OP_DONE is
set to 0), the request is ignored.
2.3 Start management
The start management block is designed to provide an anti-glitch functionality in
order to reject glitches on the input start signal and also to provide the gen_start_uc0,
gen_start_uc1, start_latch_uc0 and start_latch_uc1 signals. The main purpose of this
block is to generate the internal gen_start signals feeding the microcores starting from
the startx pins. Each microcore can be sensitive to the 6 startx pins according to the
sensitivity map defined in the register start_config_reg (104h, 124h).
This block also provides the start_latch_ucx signals; these 8-bit signals (1 for each
microcore) are used by the corresponding microcore to check which startx pin was
active when the currently ongoing actuation began. In this way each microcore can be
configured to be sensitive for up to all the 6 startx pins. While the actuation is ongoing,
it also has the ability to check the level of the startx pins in two different modes that can
be selected. The gen_start_ucx and start_latch_ucx can be generated according to two
different strategies. The strategies for the two signals can be separately selected in the
start_config_reg (104h, 124h).
Transparent mode: The gen_start_ucx is high if at least one of the starx signals is high
for which the corresponding microcore is sensitive (refer to register start_config_reg
(104h, 124h)). The start_latch_ucx signal is a living copy of the 6 startx pins for which the
channel can be sensitive.
Smart Latch mode: When a startx pin (to which the microcore is sensitive) goes high
and the start_latch_ucx is "00000000", the gen_start_ucx is set and the current startx
pin status is latched in the start_latch_ucx register. If a rising edge is detected on any
other startx pin, it is ignored. The gen_start_ucx signal goes to 0 only when the startx pin
initially latched goes low. The start_latch_ucx register is reset only by the microcode (and
this is done usually when the actuation currently ongoing is stopped by the gen_start_ucx