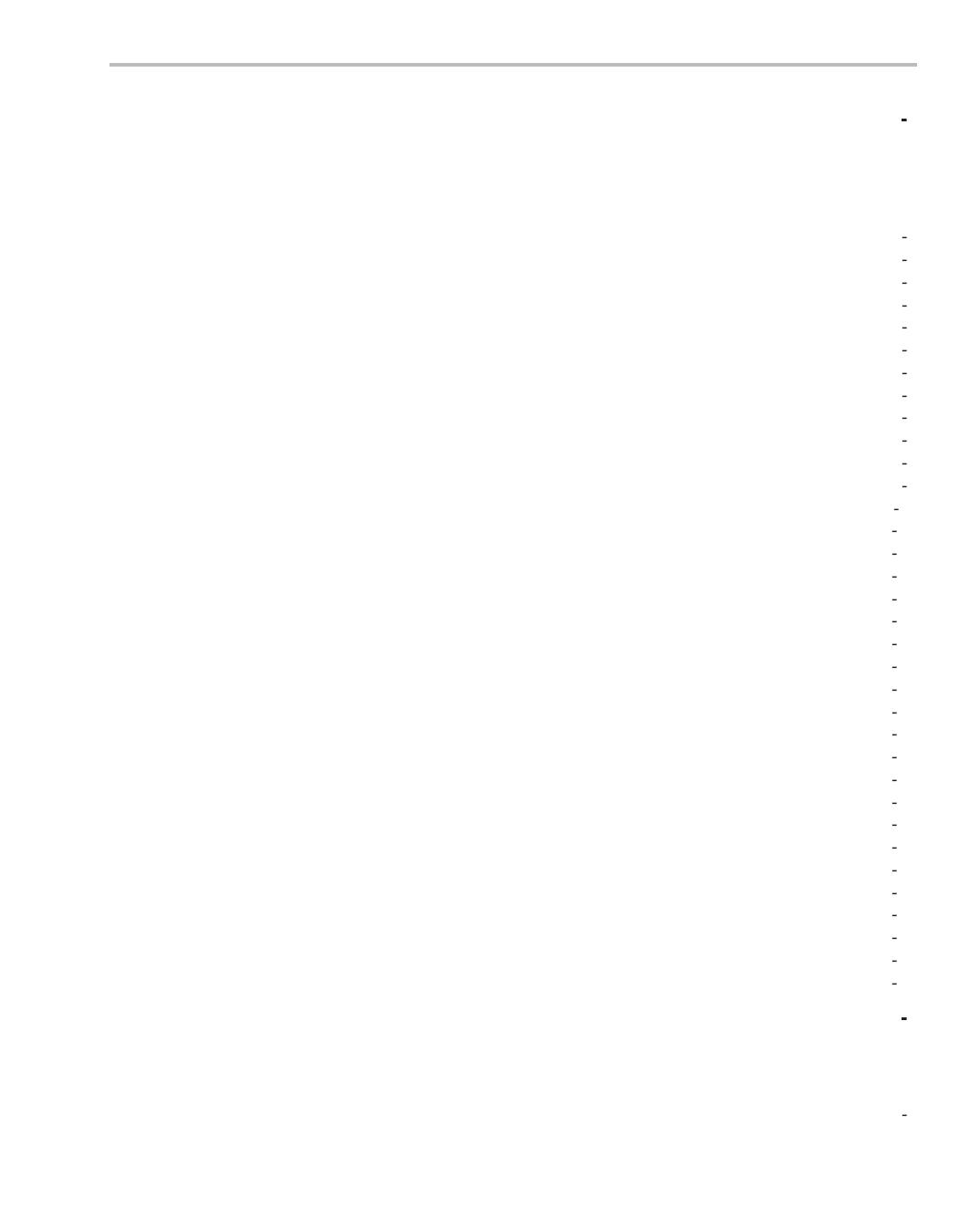
Contents
xiii
Contents
4 Runtime Environment 4 1. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
Contains technical information on how the compiler uses the TMS320C3x/C4x architecture.
Discusses memory and register conventions, stack organization, function-call conventions,
system initialization, and TMS320C3x/C4x C compiler optimizations. Provides information
needed for interfacing assembly language to C programs
4.1 Memory Model 4 2. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.1.1 Sections 4 2. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.1.2 C System Stack 4 3. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.1.3 Dynamic Memory Allocation 4 4. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.1.4 Big and Small Memory Models 4 5. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.1.5 RAM and ROM Models 4 6. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.2 Object Representation 4 7. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.2.1 Data Type Storage 4 7. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.2.2 Long Immediate Values 4 7. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.2.3 Addressing Global Variables 4 7. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.2.4 Character String Constants 4 8. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.2.5 The Constant Table 4 9. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.3 Register Conventions 4 11. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.3.1 Register Variables 4 12. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.3.2 Expression Registers 4 12. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.3.3 Return Values 4 13. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.3.4 Stack and Frame Pointers 4 13. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.3.5 Other Registers 4 14. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.4 Function Structure and Calling Conventions 4 15. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.4.1 Function Call, Standard Runtlme Model 4 16. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.4.2 Function Call, Register Argument Runtime Model 4 17. . . . . . . . . . . . . . . . . . . . . . .
4.4.3 Responsibilities of a Called Function 4 19. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.4.4 Accessing Arguments and Local Variables 4 21. . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.5 Interfacing C With Assembly Language 4 22. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.5.1 Assembly Language Modules 4 22. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.5.2 Accessing Assembly Language Variables From C 4 25. . . . . . . . . . . . . . . . . . . . . .
4.5.3 Inline Assembly Language 4 28. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.6 Interrupt Handling 4 30. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.6.1 Saving Registers During Interrupts 4 30. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.6.2 Assembly Language Interrupt Routines 4 31. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.7 Runtime-Support Arithmetic Routines 4 32. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.7.1 Precision Considerations 4 35. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.8 System Initialization 4 36. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
4.8.1 Autoinitialization of Variables and Constants 4 36. . . . . . . . . . . . . . . . . . . . . . . . . . .
5 Runtime-Support Functions 5
1. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
Describes the header files included with the C compiler, as well as the macros, functions, and
types they declare. Summarizes the runtime-support functions according to category (header),
and provides an alphabetical reference of the runtime-support functions
5.1 Runtime-Support Libraries 5 2. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .