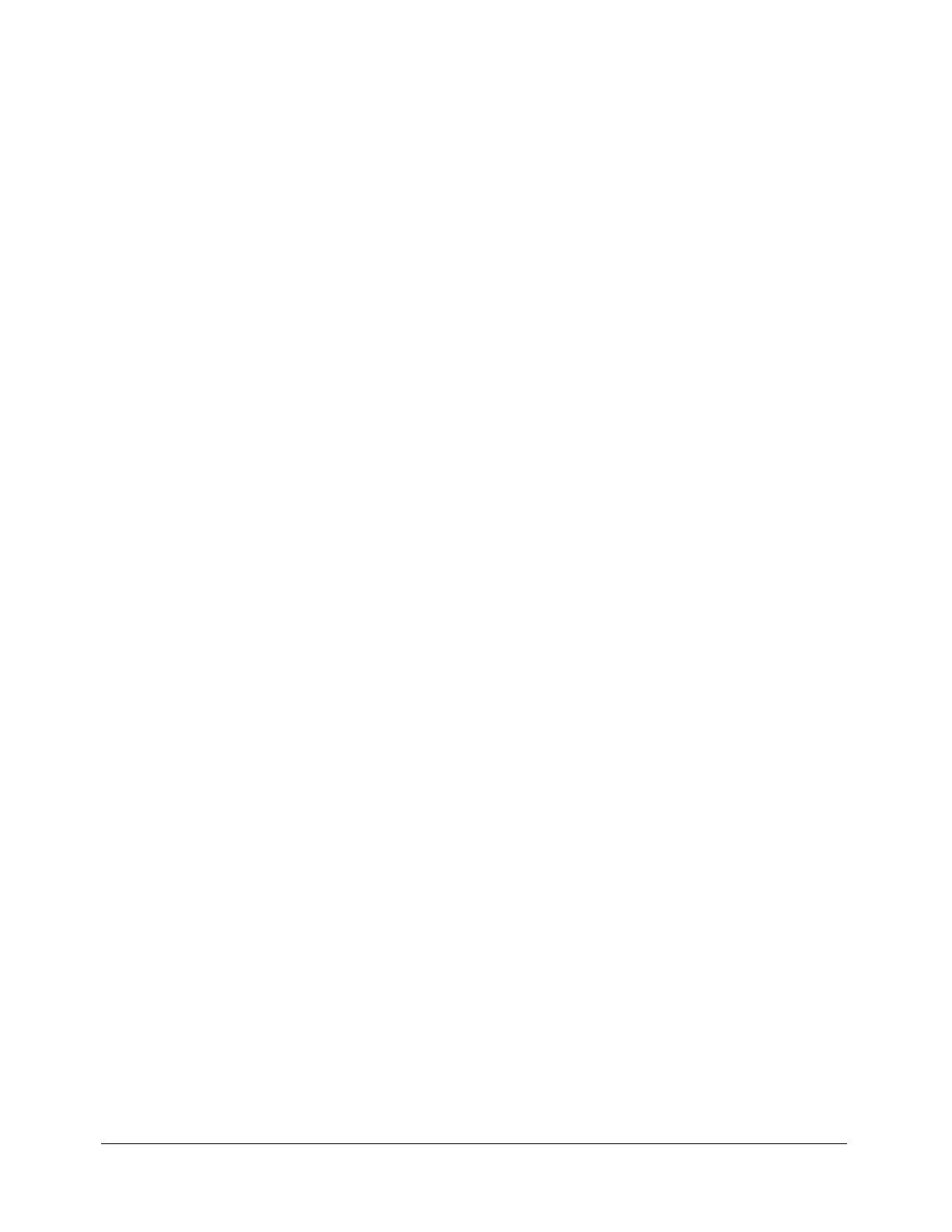
10 Creating Advanced Components
Application performance is better when you pass style properties in the initObject argument
instead of calling the
setStyle() method.
The following example creates TextInput and SimpleButton components:
function createChildren():Void {
if (text_mc == undefined)
createClassObject(TextInput, "text_mc", 0, { preferredWidth: 80,
editable:false });
text_mc.addEventListener("change", this);
text_mc.addEventListener("focusOut", this);
if (mode_mc == undefined)
createClassObject(SimpleButton, "mode_mc", 1,
{falseUpSkin:modeUpSkinName, falseOverSkin: modeOverSkinName,
falseDownSkin: modeDownSkinName });
mode_mc.addEventListener("click", this);
}
At the end of the createChildren() method, call the necessary invalidate methods
(
invalidate(), invalidateSize(), or invalidateLayout()) to refresh the screen. For more
information, see “About invalidation” on page 17.
Implementing the commitProperties() method
Flex calls the
commitProperties() method before it calls the measure() method. It lets you set
variables that are used by the
measure() method after the constructor has finished and MXML
attributes have been applied.
Flex only calls the
commitProperties() method after it calls the invalidateProperties()
method.
For example, the ViewStack container uses the
commitProperties() method to maximize
performance. When you set the
ViewStack.selectedIndex property, the ViewStack container
does not display a new page right away. Instead, it privately stores a
pendingSelectedIndex
property. When it is time for Flash Player to update the screen, Flex calls the
commitProperties(), measure(), layoutChildren(), and draw() methods. In the
commitProperties() method, the ViewStack container checks to see whether the
pendingSelectedIndex property is set, and it updates the selected index at that time.
You use the
commitProperties() method to delay processing so that Flash Player avoids doing
computationally expensive, redundant work. For example, if a script changes the
ViewStack.selectedIndex property 15 times, you would want to minimize the number of
times the display of the ViewStack container updates when the
selectedIndex property changes.
By using the commitProperties() method, you can update the pendingSelectedIndex
property 15 times, and then do the rendering only once.
This is most useful for properties that are computationally expensive to update. If setting a
property is inexpensive, you can avoid using the
commitProperties() method.
Implementing the measure() method
Generally, Flex calls the
measure() method only once, when the component is instantiated. The
component’s container sets the initial size and Flex calculates the preferred minimum and
maximum sizes. You can use the
measure() method to explicitly set the size of the component,
although Macromedia does not recommend doing this when developing components.