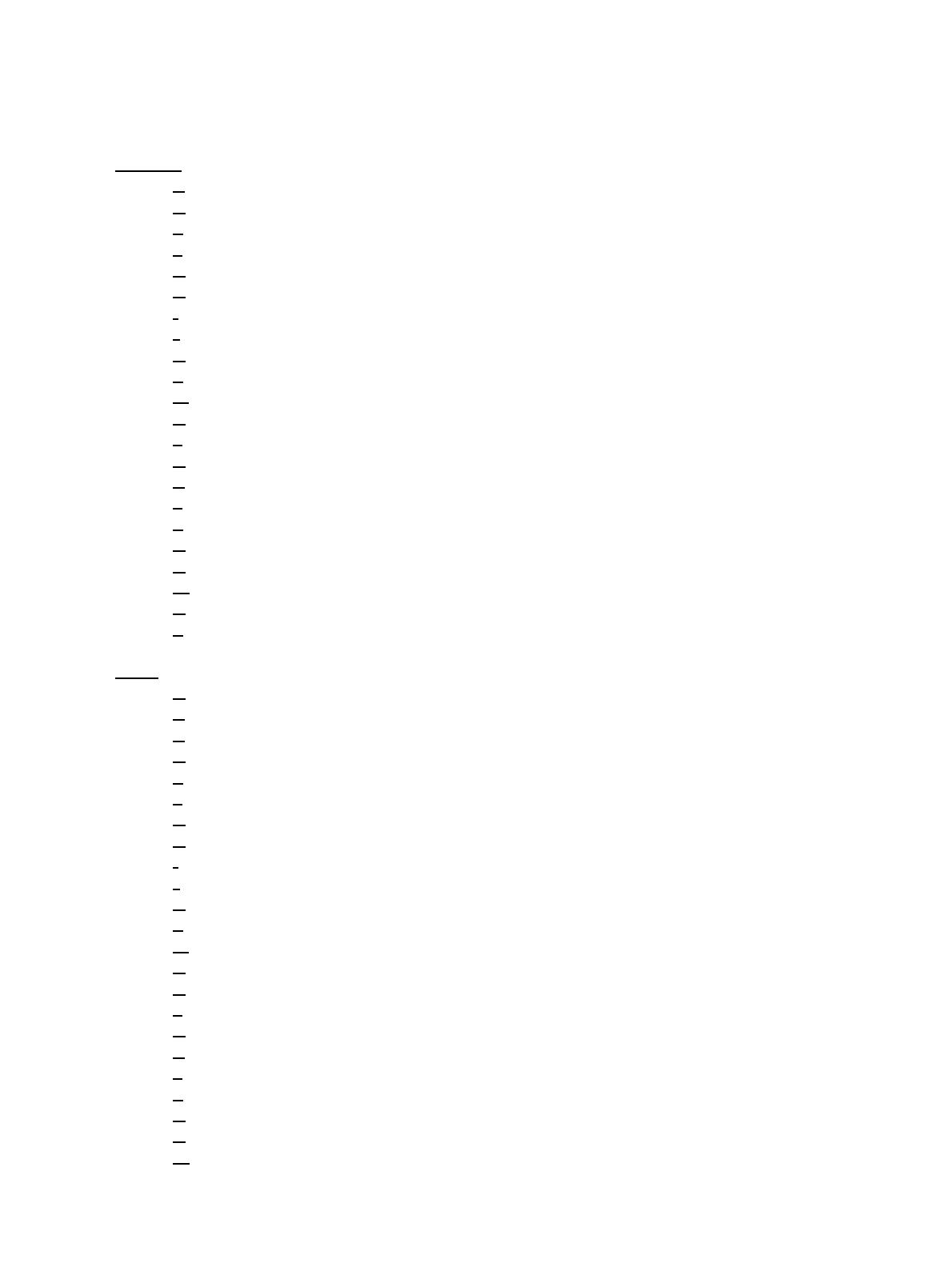
Table of Contents
Glossary
C...........................................................................................................................................................146
D...........................................................................................................................................................147
E...........................................................................................................................................................148
F...........................................................................................................................................................148
G...........................................................................................................................................................148
H...........................................................................................................................................................149
I............................................................................................................................................................149
J............................................................................................................................................................149
K...........................................................................................................................................................150
L...........................................................................................................................................................150
M..........................................................................................................................................................150
N...........................................................................................................................................................151
P...........................................................................................................................................................152
Q...........................................................................................................................................................152
R...........................................................................................................................................................152
S...........................................................................................................................................................153
T...........................................................................................................................................................153
U...........................................................................................................................................................154
V...........................................................................................................................................................154
W..........................................................................................................................................................155
X...........................................................................................................................................................155
Z...........................................................................................................................................................156
Index.................................................................................................................................................................157
A...........................................................................................................................................................157
B...........................................................................................................................................................157
C...........................................................................................................................................................158
D...........................................................................................................................................................158
E...........................................................................................................................................................158
F...........................................................................................................................................................159
G...........................................................................................................................................................160
H...........................................................................................................................................................160
I............................................................................................................................................................160
J............................................................................................................................................................161
K...........................................................................................................................................................161
L...........................................................................................................................................................161
M..........................................................................................................................................................161
N...........................................................................................................................................................161
O...........................................................................................................................................................162
P...........................................................................................................................................................162
Q...........................................................................................................................................................162
R...........................................................................................................................................................162
S...........................................................................................................................................................163
T...........................................................................................................................................................164
U...........................................................................................................................................................164
V...........................................................................................................................................................164
W..........................................................................................................................................................165
Bash Guide for Beginners
vi